To Comment or not to Comment
By Emeka Allison
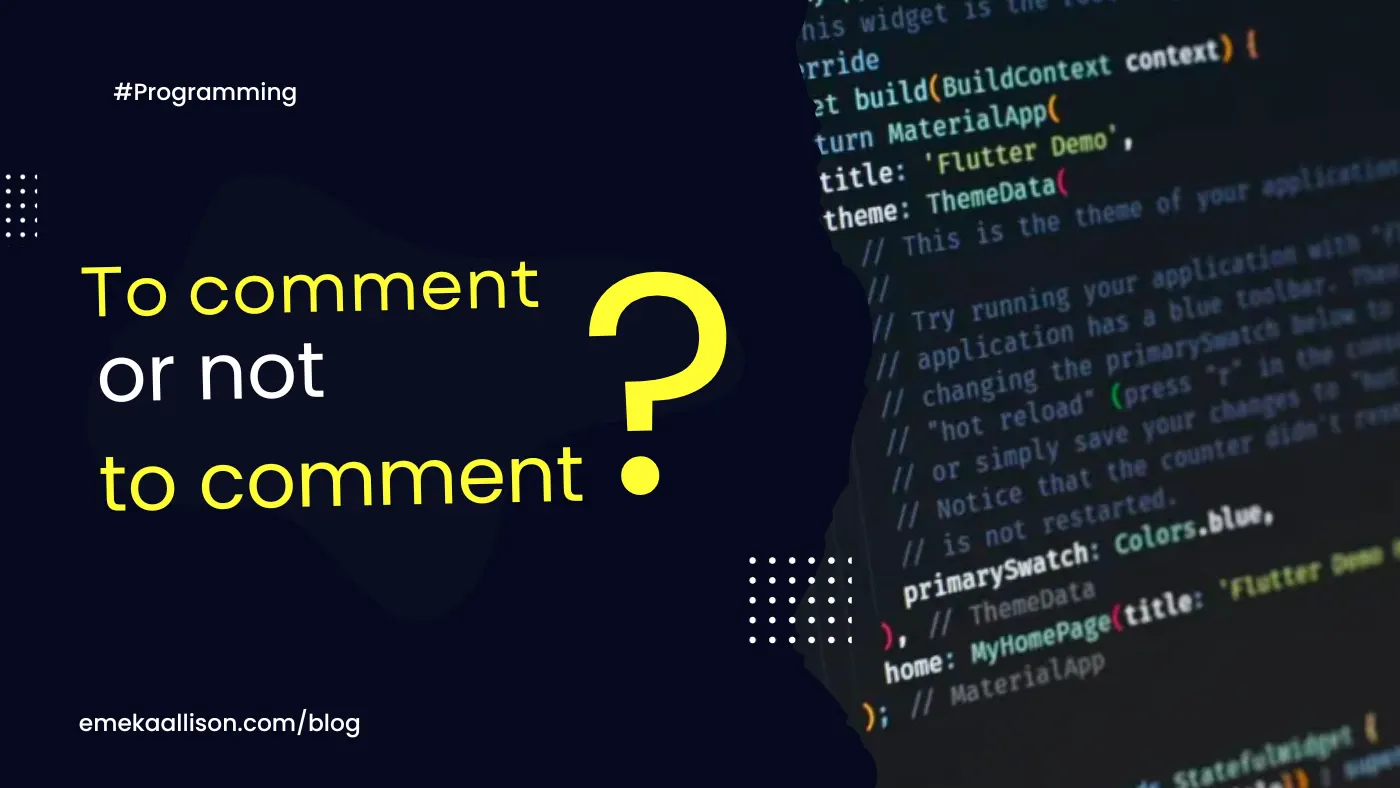
The Importance of Commenting in Code
When it comes to writing code, one of the most debated topics is whether or not to include comments. While some developers argue that comments are essential for understanding the code, others believe that well-written code should be self-explanatory. In this article, we’ll explore why commenting is important, but also emphasize the significance of code readability, proper naming conventions, and structuring your code effectively.
Code Readability
The primary goal of writing code is to create a solution that works. However, it’s equally important to ensure that your code is readable and maintainable. Readable code is easier to understand, debug, and extend. Here are some tips to improve code readability:
-
Use meaningful names: Choose descriptive names for your functions, variables, and classes. This makes it clear what each part of your code does.
-
Keep functions small: Break down large functions into smaller, more manageable pieces. Each function should have a single responsibility.
-
Consistent formatting: Follow a consistent coding style and use proper indentation. This makes your code visually appealing and easier to follow.
Naming Conventions
Proper naming conventions can significantly enhance the readability of your code. Let’s look at some examples in Python and JavaScript:
Python Example
# Bad example
def cal(x, y):
return x + y
# Good example
def calculate_sum(first_number, second_number):
return first_number + second_number
JavaScript Example
// Bad example
function fn(a, b) {
return a * b;
}
// Good example
function multiplyNumbers(firstNumber, secondNumber) {
return firstNumber * secondNumber;
}
When to Comment
While readable code is crucial, comments still play an important role. Here are some scenarios where comments are beneficial:
-
Complex logic: If your code involves complex algorithms or logic, comments can help explain the thought process behind it.
-
Workarounds: If you have to implement a workaround or a hack, it’s helpful to leave a comment explaining why it’s necessary.
-
External references: If your code relies on external resources or documentation, include comments with links or references.
Commenting Best Practices
When adding comments, follow these best practices:
-
Be concise: Keep comments short and to the point. Avoid redundant information.
-
Update comments: Ensure that comments are updated whenever the code changes. Outdated comments can be misleading.
-
Avoid obvious comments: Don’t state the obvious. For example, avoid comments like
# increment i by 1
fori += 1
.
Example of Good Commenting
Python Example
def find_prime_numbers(limit):
"""
Find all prime numbers up to a given limit.
Args:
limit (int): The upper limit to find prime numbers.
Returns:
list: A list of prime numbers up to the limit.
"""
primes = []
for num in range(2, limit + 1):
is_prime = True
for i in range(2, int(num ** 0.5) + 1):
if num % i == 0:
is_prime = False
break
if is_prime:
primes.append(num)
return primes
JavaScript Example
/**
* Find all prime numbers up to a given limit.
*
* @param {number} limit - The upper limit to find prime numbers.
* @returns {number[]} An array of prime numbers up to the limit.
*/
function findPrimeNumbers(limit) {
const primes = [];
for (let num = 2; num <= limit; num++) {
let isPrime = true;
for (let i = 2; i <= Math.sqrt(num); i++) {
if (num % i === 0) {
isPrime = false;
break;
}
}
if (isPrime) {
primes.push(num);
}
}
return primes;
}
Conclusion
In conclusion, while comments are important, they should not be a crutch for poorly written code. Prioritize code readability by using meaningful names, keeping functions small, and following consistent formatting. Use comments to explain complex logic, workarounds, and external references. By striking the right balance between readable code and helpful comments, you can create code that is both functional and maintainable.